Are you on the hunt for a simple solution to retrieve token prices using an RPC node? Look no further! In this tutorial, we will present Moralis’ Extended RPC Methods and our eth_getTokenPrice
endpoint, which allows you to obtain the price of any token with just one RPC request. Excited to see how it operates? Check out the example script below:
import fetch from 'node-fetch'; const options = { method: 'POST', headers: { accept: 'application/json', 'content-type': 'application/json' }, body: JSON.stringify({ "jsonrpc": "2.0", "id": 1, "method": "eth_getTokenPrice", "params": [ { "address": "0xA0b86991c6218b36c1d19D4a2e9Eb0cE3606eB48", "include": "percent_change" } ] }) }; fetch('YOUR_NODE_URL', options) .then(response => response.json()) .then(response => console.log(response)) .catch(err => console.error(err));
By running the above code, you will receive the price of the specified token in both the native currency of the chain and in USD. Below is an example of how the response might appear:
{ jsonrpc: '2.0', id: 1, result: { tokenName: 'USD Coin', tokenSymbol: 'USDC', tokenLogo: 'https://logo.moralis.io/0x1_0xa0b86991c6218b36c1d19d4a2e9eb0ce3606eb48_032b6f94fd2bd5af6c065def140109e9', tokenDecimals: '6', nativePrice: { value: '399015837290761', decimals: 18, name: 'Ether', symbol: 'ETH', address: '0xc02aaa39b223fe8d0a0e5c4f27ead9083c756cc2' }, usdPrice: 0.9998033934658264, usdPriceFormatted: '0.999803393465826414', '24hrPercentChange': '-0.023636130935194257', exchangeName: 'Uniswap v3', exchangeAddress: '0x1F98431c8aD98523631AE4a59f267346ea31F984', tokenAddress: '0xa0b86991c6218b36c1d19d4a2e9eb0ce3606eb48', priceLastChangedAtBlock: '20669844', possibleSpam: false, verifiedContract: true, pairAddress: '0x88e6a0c2ddd26feeb64f039a2c41296fcb3f5640', pairTotalLiquidityUsd: '165658281.27' } }
It’s that simple! Fetching token prices with an RPC node doesn’t have to be complicated. Should you desire a more comprehensive tutorial, feel free to explore our guide or refer to the eth_getTokenPrice
documentation page.
Are you ready to utilize our Extended RPC Methods? Sign up for free with Moralis today!
Overview
If you are aiming to develop cryptocurrency wallets, portfolio trackers, tax tools, or similar applications, you will most likely need access to token prices. However, using standard RPC methods for fetching token prices often necessitates multiple requests and intricate manual data aggregation. This can be both time-consuming and resource-heavy, which is why we have introduced Moralis’ Extended RPC Methods.
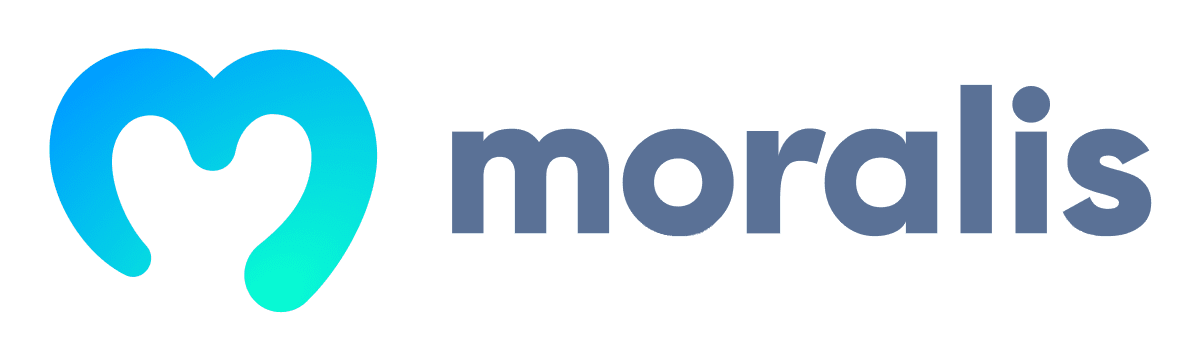
With Moralis’ Extended RPC Methods, you only need a single RPC request to get the price of any token. But how does it function? If you wish to learn how to retrieve token prices utilizing an RPC node, join us in this guide where we will break everything down for you. Let’s get started!
What are RPC Methods?
RPC stands for “Remote Procedure Call,” which refers to communication protocols that allow one software system to call and request services from software on different devices. In the realm of blockchain, RPC protocols enable decentralized applications (dapps) to communicate with blockchain networks like Ethereum, Optimism, and Base.

There are several standardized RPC protocols, with JSON-RPC being one of the most notable. It encompasses numerous predefined RPC methods like eth_getProof
that facilitate blockchain interactions and enable seamless reading and writing of on-chain data.
Below is a list of some significant methods:
eth_getTransactionByHash
: Retrieves data concerning a transaction using its hash.eth_getChainId
: Returns the current chain ID.eth_gasPrice
: Fetches the current gas price.eth_getBalance
: Retrieves the balance of a specific address.eth_getBlockNumber
: Returns the count of the most recent block.
Nevertheless, while standard RPC methods facilitate blockchain interactions, they come with several notable limitations. For example, they cannot directly obtain token prices, requiring multiple requests, often involving third-party providers to acquire this data.
To address this limitation and enhance the developer experience, we have rolled out Moralis’ next-gen RPC nodes!
Introducing Moralis’ Next-Generation RPC Nodes – The Simplest Way to Retrieve Token Prices
Moralis stands out as a leading RPC node provider, offering next-generation nodes that streamline your development process. With our user-friendly point-and-click interface, you can effortlessly set up RPC nodes for all major chains, including Ethereum, Optimism, Base, Polygon, BNB Smart Chain (BSC), and more.

What makes our RPC nodes exceptional?
- Speed: We set the standard for speed, with response times as low as 70 ms.
- Reliability: Moralis’ nodes offer an impressive 99.9% uptime, ensuring you receive all the data you need without issues.
- Extended RPC Methods: Through our Extended RPC Methods, you can easily fetch decoded, human-readable data using RPC-style requests.
Enjoy the advantages of next-generation RPC nodes with rapid response times, unparalleled reliability, and our powerful Extended RPC Methods!
Extended RPC Methods
Moralis’ Extended RPC Methods enable you to effortlessly acquire decoded, human-readable data through RPC-style requests. With a single call, you can obtain NFT balances, ERC-20 balances, decoded wallet history, metadata, token prices, and more. Consequently, using Moralis allows you to enhance your development experience and build dapps more efficiently and swiftly.

Here is a list of all available methods:
eth_getTokenPrice
: Retrieve the price of any ERC-20 token.eth_getNFTBalances
: Fetch the NFT balance of a wallet.eth_getNFTCollections
: Obtain all collections held by a wallet.eth_getTransactions
: Query the native transactions of a wallet.eth_getDecodedTransactions
: Access a wallet’s complete transaction history.eth_getTokenBalances
: Query the ERC-20 balances of a wallet.eth_getTokenMetadata
: Retrieve the metadata of an ERC-20 token.
In summary, the provided methods grant you seamless access to decoded, human-readable data via RPC-style requests.
eth_getTokenPrice
– Retrieve Token Prices with One RPC Call
With our eth_getTokenPrice
method, obtaining token prices has never been easier. A single RPC request delivers the price of any token in both the chain’s native cryptocurrency and USD. Moralis has simplified the process of accessing token prices using an RPC node.

So how does the eth_getTokenPrice
method work? What does a response entail? If you seek answers to these questions, continue reading as we guide you through a straightforward tutorial on how to acquire token prices via an RPC node in three easy steps!
3-Step Tutorial: How to Retrieve Token Prices with an RPC Node
We will now guide you through the process of obtaining token prices using an RPC node. Thanks to the ease of our Extended RPC Methods, you can gather this data in three straightforward steps:
- Sign Up with Moralis & Create a Node
- Develop a Script Calling
eth_getTokenPrice
- Execute the Code
Before proceeding, ensure you address a couple of prerequisites!
Prerequisites
If you have not done so already, please prepare the following before moving forward:
Step 1: Sign Up with Moralis & Create a Node
Create a Moralis account by clicking the “Start for Free” button in the upper right corner:

Log in, find the “Nodes” tab, and click “+ Create Node”:

Select “Ethereum,” “Mainnet,” and hit “Create Node”:

Copy and store one of your node URLs, as you will need it in the next step:

Step 2: Develop a Script Calling eth_getTokenPrice
Create a new folder in your favorite IDE and initialize a project using the terminal command below:
npm init
Install the required dependencies by executing the commands below in your terminal:
npm install node-fetch --save npm install moralis @moralisweb3/common-evm-utils
Add ”type”: ”module”
to your ”package.json” file:

Create a new “index.js” file and incorporate the following code:
import fetch from 'node-fetch'; const options = { method: 'POST', headers: { accept: 'application/json', 'content-type': 'application/json' }, body: JSON.stringify({ "jsonrpc": "2.0", "id": 1, "method": "eth_getTokenPrice", "params": [ { "address": "0xA0b86991c6218b36c1d19D4a2e9Eb0cE3606eB48", "include": "percent_change" } ] }) }; fetch('YOUR_NODE_URL', options) .then(response => response.json()) .then(response => console.log(response)) .catch(err => console.error(err));
Substitute YOUR_NODE_URL
with the URL you copied in the previous step and alter the address
parameter as needed:

Step 3: Execute the Code
Run the command below in your project’s root folder to execute the script:
node index.js
As a result, you will get the price of the specified token quoted in both the native currency of the chain and in USD. Additionally, the response will include token logos, symbols, price variations over time, and much more. Here is an example of how it might appear:
{ jsonrpc: '2.0', id: 1, result: { tokenName: 'USD Coin', tokenSymbol: 'USDC', tokenLogo: 'https://logo.moralis.io/0x1_0xa0b86991c6218b36c1d19d4a2e9eb0ce3606eb48_032b6f94fd2bd5af6c065def140109e9', tokenDecimals: '6', nativePrice: { value: '399015837290761', decimals: 18, name: 'Ether', symbol: 'ETH', address: '0xc02aaa39b223fe8d0a0e5c4f27ead9083c756cc2' }, usdPrice: 0.9998033934658264, usdPriceFormatted: '0.999803393465826414', '24hrPercentChange': '-0.023636130935194257', exchangeName: 'Uniswap v3', exchangeAddress: '0x1F98431c8aD98523631AE4a59f267346ea31F984', tokenAddress: '0xa0b86991c6218b36c1d19d4a2e9eb0ce3606eb48', priceLastChangedAtBlock: '20669844', possibleSpam: false, verifiedContract: true, pairAddress: '0x88e6a0c2ddd26feeb64f039a2c41296fcb3f5640', pairTotalLiquidityUsd: '165658281.27' } }
That’s it! You now know how to retrieve token prices using an RPC node!
Use Cases for Token Prices
Token prices are essential in numerous dapps. Here are three key examples:
- Web3 Wallets: Web3 wallets usually enable users to buy and sell cryptocurrencies. To offer a seamless experience, these platforms require accurate token prices.
- Portfolio Trackers: Portfolio trackers provide users with an overview of their digital assets. To deliver an accurate performance snapshot, they must access current cryptocurrency prices.
- Tax Platforms: Tax software necessitates both historical and current token prices to create precise tax reports.

These are merely a few instances. Token price data is also critical for the development of decentralized exchanges (DEXs), token analytics platforms, and more.
Beyond Token Prices with an RPC Node – Exploring Other Extended RPC Methods
Now that you understand how to retrieve token prices using a node, let’s take a closer look at some of our other Extended RPC Methods. Specifically, we’ll examine the following three:
eth_getTransactions
eth_getTokenBalances
eth_getNFTBalances
eth_getTransactions
The eth_getTransactions
method allows you to effortlessly retrieve wallet transactions through RPC nodes. You only need one RPC request to obtain the complete native transaction history for a specified wallet. Below is an example of how this works:
import fetch from 'node-fetch'; const options = { method: 'POST', headers: { accept: 'application/json', 'content-type': 'application/json' }, body: JSON.stringify({ "jsonrpc": "2.0", "id": 1, "method": "eth_getTransactions", "params": [ { "address": "0xd8dA6BF26964aF9D7eEd9e03E53415D37aA96045", "limit": 100, } ] }) }; fetch('YOUR_NODE_URL', options) .then(response => response.json()) .then(response => console.log(response)) .catch(err => console.error(err));
Upon executing the above code, you will receive a list of past native transactions for the specified wallet. Additionally, each transaction is enriched with address labels, gas prices, and more details. Here is a sample response:
{ //... result: [ { hash: '0xd89b02f289a08ae7b2feead06031fec20777bad8b73fc8d853f9040bc423a6c7', nonce: '0', transaction_index: '142', from_address: '0xda74ac6b69ff4f1b6796cddf61fbdd4a5f68525f', from_address_label: '', to_address: '0xdac17f958d2ee523a2206206994597c13d831ec7', to_address_label: 'Tether USD (USDT)', value: '0', gas: '207128', gas_price: '17020913648', input: '0xa9059cbb00000000000000000000000028c6c06298d514db089934071355e5743bf21d6000000000000000000000000000000000000000000000000000000017a1df1700', receipt_cumulative_gas_used: '8270587', receipt_gas_used: '41309', receipt_contract_address: null, receipt_root: null, receipt_status: '1', block_timestamp: '2023-01-22T15:00:11.000Z', block_number: '16463098', block_hash: '0x2439330d0a282f9a6464b0aceb9f766ac4d7b050c048b4a1322b48544c61e01d', transaction_fee: '0.000703116921885232' }, //... ] } }
eth_getTokenBalances
With the eth_getTokenBalances
endpoint, you can easily acquire ERC-20 token balances using RPC nodes. A single call is all you need. Here’s a practical example of this method:
import fetch from 'node-fetch'; const options = { method: 'POST', headers: { accept: 'application/json', 'content-type': 'application/json' }, body: JSON.stringify({ "jsonrpc": "2.0", "id": 1, "method": "eth_getTokenBalances", "params": [ { "address": "0xcB1C1FdE09f811B294172696404e88E658659905", } ] }) }; fetch('YOUR_NODE_URL', options) .then(response => response.json()) .then(response => console.log(response)) .catch(err => console.error(err));
Executing the above endpoint will yield the ERC-20 balances for the specified wallet, complete with logos, decimals, thumbnails, spam indicators, and additional information for each token. Below is how the response may appear:
{ //... result: [ { token_address: '0xc02aaa39b223fe8d0a0e5c4f27ead9083c756cc2', name: 'Wrapped Ether', symbol: 'WETH', decimals: 18, logo: 'https://logo.moralis.io/0x1_0xc02aaa39b223fe8d0a0e5c4f27ead9083c756cc2_a578c5277503e547a072ae32517254ca', thumbnail: 'https://logo.moralis.io/0x1_0xc02aaa39b223fe8d0a0e5c4f27ead9083c756cc2_a578c5277503e547a072ae32517254ca', balance: '10000000000000000', possible_spam: false, verified_contract: true, total_supply: '2746607222348759943423350', total_supply_formatted: '2746607.22234875994342335', percentage_relative_to_total_supply: 3.64085549569e-7 }, //... ] }
eth_getNFTBalances
The eth_getNFTBalances
method allows you to easily obtain NFT balances with a single RPC call. Integrate NFT balances into your dapps without hassle. Below is a sample script demonstrating how it operates:
import fetch from 'node-fetch'; const options = { method: 'POST', headers: { accept: 'application/json', 'content-type': 'application/json' }, body: JSON.stringify({ "jsonrpc": "2.0", "id": 1, "method": "eth_getNFTBalances", "params": [ { "address": "0xDc597929101c2DE50c97D43C8EA3A372Bf55fdc0", "limit": 10, } ] }) }; fetch('YOUR_NODE_URL', options) .then(response => response.json()) .then(response => console.log(response)) .catch(err => console.error(err));
When you call the eth_getNFTBalances
endpoint, you will receive the NFT balances of the specified wallet, along with enriched details such as addresses, collection data, metadata, and more:
{ jsonrpc: '2.0', id: 1, result: { //... result: [ { amount: '1', token_id: '1919', token_address: '0xbd3531da5cf5857e7cfaa92426877b022e612cf8', contract_type: 'ERC721', owner_of: '0xdc597929101c2de50c97d43c8ea3a372bf55fdc0', last_metadata_sync: '2024-08-25T23:35:51.384Z', last_token_uri_sync: '2024-08-25T23:35:50.893Z', metadata: '{"attributes":[{"trait_type":"Background","value":"Mint"},{"trait_type":"Skin","value":"Olive Green"},{"trait_type":"Body","value":"Turtleneck Pink"},{"trait_type":"Face","value":"Eyepatch"},{"trait_type":"Head","value":"Wizard Hat"}],"description":"A collection 8888 Cute Chubby Pudgy Penquins sliding around on the freezing ETH blockchain.","image":"ipfs://QmNf1UsmdGaMbpatQ6toXSkzDpizaGmC9zfunCyoz1enD5/penguin/1919.png","name":"Pudgy Penguin #1919"}', block_number: '19754671', block_number_minted: null, name: 'PudgyPenguins', symbol: 'PPG', token_hash: 'cbd8bd0901f422afb88e76615e3d2a1a', token_uri: 'https://ipfs.moralis.io:2053/ipfs/bafybeibc5sgo2plmjkq2tzmhrn54bk3crhnc23zd2msg4ea7a4pxrkgfna/1919', minter_address: null, verified_collection: true, possible_spam: false, collection_logo: 'https://i.seadn.io/gae/yNi-XdGxsgQCPpqSio4o31ygAV6wURdIdInWRcFIl46UjUQ1eV7BEndGe8L661OoG-clRi7EgInLX4LPu9Jfw4fq0bnVYHqg7RFi?w=500&auto=format', collection_banner_image: 'https://i.seadn.io/gcs/files/8a26e3de0f309089cbb1e5ab969fc0bc.png?w=500&auto=format' }, //... ] } }
Visit our official Extended RPC Methods documentation page to discover the remaining methods!
Discovering Moralis’ Web3 API Suite
In addition to our Extended RPC Methods, we also provide a comprehensive suite of Web3 APIs. Prominent examples include the Wallet API, Token API, Streams API, NFT API, and many more. These APIs offer deeper and richer data, simplifying the development process for everything from Web3 wallets to games!
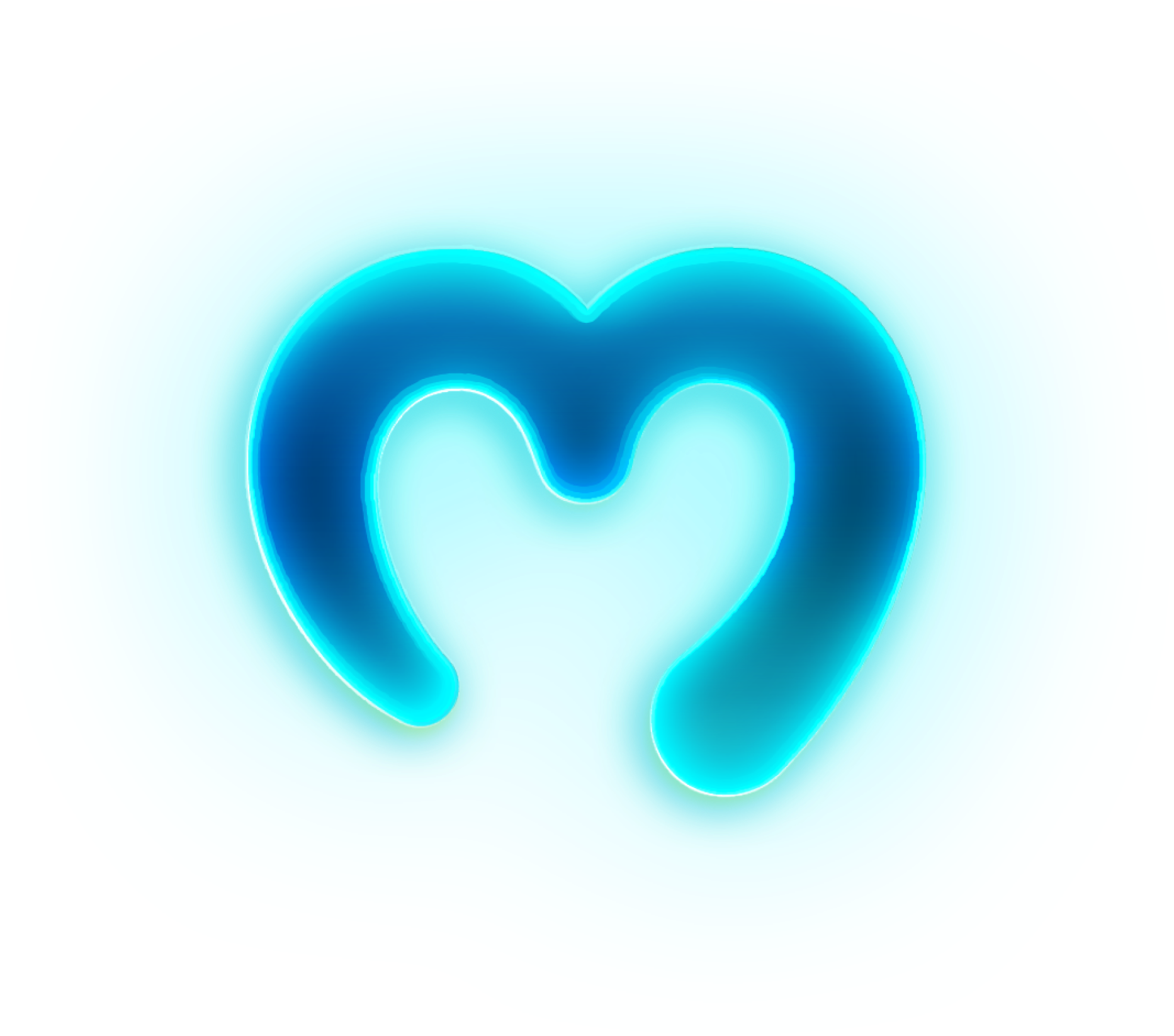
What are the advantages of utilizing our APIs?
- Comprehensive: Our APIs provide more data with fewer calls. Obtain a wallet’s complete history, token balances with prices, updated NFT metadata, and more with just one request.
- Cross-Chain: Moralis’ APIs are fully cross-chain, ensuring feature parity across all major networks, including Ethereum, Polygon, Optimism, BSC, Base, and others.
- Secure & Reliable: We proudly hold a SOC 2 Type 2 certification, underscoring our commitment to maintaining enterprise-grade security and reliability.
Now, let’s delve into some of our APIs in greater detail!
Web3 APIs
Our suite of Web3 APIs encompasses various use case-specific interfaces. Here are three key examples:
- Wallet API: Retrieve a wallet’s complete transaction history, token balances, net worth, profitability, token approvals, and much more with just a few lines of code.
- Token API: Get token prices, metadata, transactions, balances, and more with just one request.
- NFT API: Query NFT balances, current metadata, prices, etc., with a single API call.
Visit our Web3 API page to explore all our industry-leading APIs!
Summary: How to Retrieve Token Prices with an RPC Node
Retrieving token prices through traditional RPC methods tends to require multiple requests and extensive manual data aggregation. This process can be both time-consuming and resource-intensive. Luckily, you can bypass these hassles and find token prices using an RPC node in a single call with Moralis’ Extended RPC Methods!
Using our Extended RPC Methods, you can effortlessly retrieve decoded, human-readable data with RPC-style requests. Access token balances, prices, NFT balances, and much more with single calls.
To emphasize the simplicity of this feature, check out the example script below demonstrating how to obtain token prices with an RPC node:
import fetch from 'node-fetch'; const options = { method: 'POST', headers: { accept: 'application/json', 'content-type': 'application/json' }, body: JSON.stringify({ "jsonrpc": "2.0", "id": 1, "method": "eth_getTokenPrice", "params": [ { "address": "0xA0b86991c6218b36c1d19D4a2e9Eb0cE3606eB48", "include": "percent_change" } ] }) }; fetch('YOUR_NODE_URL', options) .then(response => response.json()) .then(response => console.log(response)) .catch(err => console.error(err));
By invoking this script, you will receive the price of the specified token in both USD and the chain’s native currency. Here’s an illustration of what the response could look like:
{ jsonrpc: '2.0', id: 1, result: { tokenName: 'USD Coin', tokenSymbol: 'USDC', tokenLogo: 'https://logo.moralis.io/0x1_0xa0b86991c6218b36c1d19d4a2e9eb0ce3606eb48_032b6f94fd2bd5af6c065def140109e9', tokenDecimals: '6', nativePrice: { value: '399015837290761', decimals: 18, name: 'Ether', symbol: 'ETH', address: '0xc02aaa39b223fe8d0a0e5c4f27ead9083c756cc2' }, usdPrice: 0.9998033934658264, usdPriceFormatted: '0.999803393465826414', '24hrPercentChange': '-0.023636130935194257', exchangeName: 'Uniswap v3', exchangeAddress: '0x1F98431c8aD98523631AE4a59f267346ea31F984', tokenAddress: '0xa0b86991c6218b36c1d19d4a2e9eb0ce3606eb48', priceLastChangedAtBlock: '20669844', possibleSpam: false, verifiedContract: true, pairAddress: '0x88e6a0c2ddd26feeb64f039a2c41296fcb3f5640', pairTotalLiquidityUsd: '165658281.27' } }
Congratulations! You are now equipped to retrieve token prices using an RPC node!
If you found this tutorial helpful, consider exploring additional articles on the Moralis blog. For example, delve into the intricacies of our Blockchain Address Labeling API.
And if you’re keen on utilizing our Extended RPC Methods, don’t forget to sign up with Moralis. You can register for free and gain immediate access to all our cutting-edge tools!